The Secrets of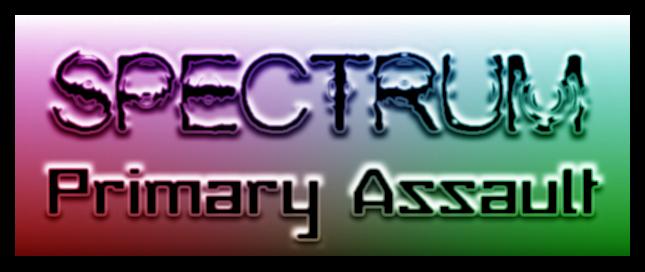
(adapted from cs248 report) |
Procedural and physically-based modeling
The area where the game excels most memorably is in the realm of physics-based
modeling.
Hydrodynamics (water physics)
The physics of the water surface's movement was one of the primary focuses of the game. We wanted not only to make it a visually appealing effect, but also an integral part of the gameplay. The water's surface is a surprisingly low-polygon mesh (around 3000 polygons) with vertices spaced out evenly
in a grid over the ground plane. Each vertex has a position, velocity, and viscosity. The idea is to model the cohesive forces between adjacent particles of liquid. When one vertex is disturbed, it creates an imbalance in the system. At every time step, each vertex examines the difference in its height position and the height of its neighboring vertices, and adjusts its velocity to compensate for the attractive forces. Graphically, it was important to adjust the lighting normals at each time step to make the surface appear smooth (and not like a jagged height map). There were a number of papers and demos online with ideas we used about how to accomplish water physics.
Ripples
A single ripple is accomplished by giving the vertex nearest to the disturbance an initial velocity. Once the dynamics of the whole system is modeled, the rippling effect will propagate throughout the system. Shoot down a guardian to observe a single ripple.
Continuous perturbations
These were achieved essentially by creating a sequence of ripples (velocity perturbations) in such a way that would simulate a continuous disturbance. Observe Ecclipsor's complex interactions with the water as he submerges/emerges to see this in action. Also, try spraying the water in a continuous way.
Color staining
This was accomplished using additive color. The color of the stain is averaged with the color of the water's surface, and each quad is colored by interpolating the color of its four vertices. With smooth shading turned on, this creates a very soft, anti-aliased effect. Try painting circular streaks on the water's surface. Ecclipsor's black sludge trail is another example of color staining.
Geysers
Realistically a geyser like those generated by the Geyser Bombs could not exist with the realm of a normal hydrodynamics system. They create an imbalance so pronounced that the water surface goes
berzerk. To remedy this, we added a great deal of special case code to
treat geysers as relatively self-contained phenomena that had a reduced effect on neighboring water particles. Try shooting bombs to observe geysers.
Vortexes
Vortexes are essentially the same problem as geysers (but upside-down). One main difference is that they seep down gradually, and must maintain their shape for arbitrary amounts of time. Observe Ecclipsor emerging from the vortex in the beginning of the game.
Terrain Generation
We used the diamond square algorithm to fractally generate our terriain. It is basically a 2D interpretation of the 1D midpoint displacement algorithm. For smooth performance at run-time, we chose to generate 10 random compatible (meaning that the connecting edges were all seeded to match up) terrain maps which would be randomly placed on the map as the player progressed through the game. These fractals were also seeded to guarantee that we had a valley in the center where our game takes place. Though it is possible to get very detailed fractal terrains by doing many recursive iterations, it is difficult to draw all those polygons. Instead, we used the same algorithm at startup to generate a few very detailed fractals, render and light them and then read them back as a texture maps. These maps were also seeded to be compatible, so we would be able to permute both the terrain itself and also the bitmap textured onto it. In addition, to add a higher level of realism, we also added multitexturing to give the terrain sharp looking features even very close up. The tutorial and writeup by Paul Martz at GameProgrammer.com were very useful for getting started with terrain generation. The concept of texture mapping a more highly developed bitmapped lit fractal terrain onto a lower poly surface was suggested there.
Simulated Dynamics
Fluid Pressure Dynamics
The liquid emitted from the Spectral Cannon uses a different kind of physics from the liquid on the ground. The stream is modeled as a particle system, whose particles use parameters such as the player's orientation and velocity, the amount of remaining fluid in the reservoir, and randomized "sputter" factors to simulate a streamlike flow of droplets. The model was tweaked using Mario's water cannon in Super Mario Sunshine (as well as a garden
hose) as benchmarks. Try spraying the water and watch the stream's trajectory. Make sure to spray until the cannon sputters out.
"Billboarding" is used to allow 2D sprites to be used to
represent water droplets.
Rope Physics
From the beginning of our work on the game we wanted to find a way to connect the color changes on the surface of the
water to the color of the energy shot by our plane. It seemed only naturally to do that by way of a rope (the "Spectral Siphon"). The physics of the rope is fairly straightforward. Masses are connected by springs which apply force to one another based on their positions and the spring constant (k) of the spring. Gravity, a spring friction k, and an air friction k are also applied. These forces were applied using the Euler method. In order to connect this rope to the plane it was necessary to fix an anchor point to its underbelly and then calculate incremental velocities at each frame to apply to the mass anchored at that point. The rest of the masses followed along with realistic physics, simulating the appearance of the rope. In order to prevent it from swinging around the plane like crazy, we set the spring friction constant and constant of air friction to much higher values than they would normally be. The physics tutorials at Nehe were useful starting points for learning about physical simulations.
Each of Ecclipsor's segments also has a tentacle, which is modeled kinematically using the same rope physics model as the Spectral Siphon.
Dampened Spring Systems
Spring physics occurs everywhere in SPECTRUM, which gives the game its fluid, continuous feel.
Camera's position and orientation
The camera's movements are attached to the player using springs. You'll notice the camera's motions are never jittery of stuttered. The camera's focus tends towards the ship and the space in front of the ship, but uses dampened springs to gradually ease into those positions and orientations.
Cross-hairs and turret
Rather than attaching the cross-hairs to the *position* of the mouse, which gives a very cursor-like feel, we attach it to the *velocity* of the mouse. This way, the motion feels more continuous. The turret, in turn, is attached to the cross-hairs using a spring system. (When you move the mouse around quickly, you notice a slight lag between where the cross-hairs are and where the turret points).
This allows for more precise control of the cannon's stream and more realistic turret movements - otherwise the turret would flail around cartoonishly fast.
Players movements
The ship's translational movements, as well as its yaw, pitch, and roll, are modeled using six springs (one for each degree of freedom). Rather than simply incrementing position values discretely when the keyboard is pressed, we achieve very fluid motion by attaching the keyboard to various parameters, and slowly easing into those target values using dampened spring systems.
Enemy's movements
The swarms of guardian enemies have somewhat scripted paths, but these paths are only loose guidelines. Springs are used to ease these enemies onto their paths when they're disturbed.
Ecclipsor's segments
Ecclipsor's undulatory motion is accomplished also using springs. The position of each segment is attached to the position of its parent segment using a spring. Ecclipsor's head is its only component that is explicitly controlled.
Water color transitions
Even the way in which the color of the water's surface changes is mapped using springs. You'll notice that the water's color transitions smoothly from one primary to the next (by interpolating color values). The alternative is a very discrete and unnatural-looking color change.
Collision detection and resolution
There are a number of combinations of objects for which we do collision detection and resolution. We perform collision checking using bounding spheres and triangle-to-triangle intersections (the collision model for any ship is simply a triangle, since this is a good approximation of the model).
Player-to-Enemy
This is perhaps the most visually appealing collision. We use the principle of conservation of momentum to resolve the collision. Try getting hit in various directions and you'll notice that the ship it thrown back in a way that reflect the nature of the collision (e.g., if the enemy flies at you from the right, you'll fly left with a proportional velocity). There is also a random jitter translation applied to the player's ship after the collision. All of these factors also visibly effect the Spectral Siphon (rope tail). Finally, the ship restores to it's initial position using spring physics. The overall effect is quite compelling.
Player's stream-to-Enemy
This is simple a point to bounding sphere intersection (since it occurs at such a high frequency, it had to be a simple computation).
Enemy's pulse-to-Player
This is the same procedure as above
Enemy-to-Water
The detection for collision between the enemies and the water is a simple y-value check. However, the resolution involves using the direction and orientation of the impact of the collision to create a ripple in the water of magnitude proportional to the force of impact.
Enemy-to-Geyser
This refers to the collision detection of the geysers created using Geyser Bombs with the enemies. The resolution is done by constantly applying forces away from the geyser at every timestep. This creates a large continuous impact (as in an explosion).
Artificial Intelligence
The overall path of the guardians is somewhat scripted, but the reactions to the player's motions and recovery from the player's attacks all use adaptive AI flocking strategies. The overall swarm has its own behavior, and each individual in the swarm is aware of it's own position in the flock. Moving around from the left to right, you'll notice that the entire swarm track your motions and each ship adjusts it's flight path to stay somewhat in front of you. The ships flock in a circular path, and are evenly spread out in the circle. As you destroy enemies, the ships will reconfigure themselves to retain a circular path, slowly spreading themselves out evenly in a circle to compensate for their fallen comrade. The aiming of the kamikazee attacks is tweaked such that it is not perfectly precise. Roughly one third of the kamikazee attack will hit the player if it is standing still. The same is true for their pulse projectiles. They'll recover from their kamikazee attack and reform in their flock using the same flocking strategies.
On-screen control panel
The most advanced aspect of our graphical user interface is the 3D cross-hairs. The cross hairs are 3D objects in world space that project onto the closest surface the mouse points to. This is accomplished using the Z-buffer at the cursor position's coordinates (which is computed already by the OpenGL pipeline), and then doing a bounding box collision detection with the world space "unprojection" to determine which object is selected. The circle drawn before the cross hairs is also in world space, and gives a sense of depth perception to give some idea for how far back the cross hairs are pointing). Invisible backplanes are used extensively to allow the cross hairs to focus on enemies more easily.
The "locking box" is another advanced feature. It is a world-space bounding box that encompasses the last enemy locked onto by the cross hairs. Above it, fading RGB health bars are rendered to show the remaining life energy values of the selected enemy.
The radar is an orthographic re-rendering of all the living characters in the game as OpenGL primitives (the player, the power-ups, the guardians, and the segments of Ecclipsor). The color of the object in the radar represents the color of that object's remaining life energy.
The player's life and the amount of the fluid left in the Spectral Cannon are represented using 3D rotation sphere objects.
The power-ups are selectable re-renderings of their in-game models.
|
Borrowed Work
Models
We use only two models in the game, both rather low-polygon models (a ship and a missile). This enables us to keep load times low, memory small, and disk size minimal. The player and all the enemies (except the missile enemies) use the same basic model (scaled and lit differently). Even Ecclipsor's skeleton uses the plane model!
Music
The music we chose as our background music is a MIDI version of Metallica's "Blackened", which, for a number of reasons, we felt was an appropriate soundtrack to our game.
|
Return to main page for Spectrum:
Primary Assault.
|